Power of Python Arrays:
What is Python Array :
The programming language Python is strong and flexible; it provides a range of data structures for effective data handling and manipulation. One example of such a data structure is the Python array, which is an essential tool for methodically storing and retrieving elements. The features, applications, and benefits of Python arrays will be thoroughly examined in this blog post.
Understanding Python Arrays:
A Python array is essentially a collection of elements of the same type arranged in a sequential order. Arrays, unlike lists, are more memory efficient and provide faster access to elements due to their fixed size. The array module in Python provides a dedicated class to work with arrays, allowing for efficient storage of numeric data.
Declaration and Initialization:
To create In conclusion, Python arrays are a powerful tool for handling and manipulating data efficiently. With their fixed-size, homogeneous elements, and dedicated array module, they offer advantages in terms of performance and memory usage. Whether you’re working on numerical computations or memory-constrained environments, understanding and utilizing Python arrays can significantly enhance your programming capabilities an array in Python, simply import the array module. The syntax for creating an array consists of specifying the data type and initializing it with a sequence of values. For example:
from array import array
my_array = array('i', [1, 2, 3, 4, 5])
Here, ‘i’ denotes the data type (integer) of the array, and the list [1, 2, 3, 4, 5]
represents the initial values.
Key Characteristics:
- Homogeneous Elements: Python arrays contain elements of the same data type, ensuring uniformity and efficient memory usage.
- Fixed Size: Unlike lists, arrays have a fixed size upon creation. This characteristic provides a performance boost for specific use cases.
Use Cases:
- Numeric Operations: Python arrays are particularly useful when dealing with numeric data, such as in scientific computing or data analysis.
- Memory Efficiency: Applications that require memory optimization, like embedded systems or low-level programming, benefit from the fixed-size nature of arrays.
- Performance: Due to their fixed size and homogeneous elements, arrays offer faster access to elements compared to lists, making them suitable for performance-critical applications.
Advantages of Python Arrays:
- Efficiency: Arrays provide faster access times and reduced memory overhead compared to lists, making them ideal for scenarios where performance is crucial.
- Type Safety: The use of a specified data type ensures type safety, reducing the chances of runtime errors.
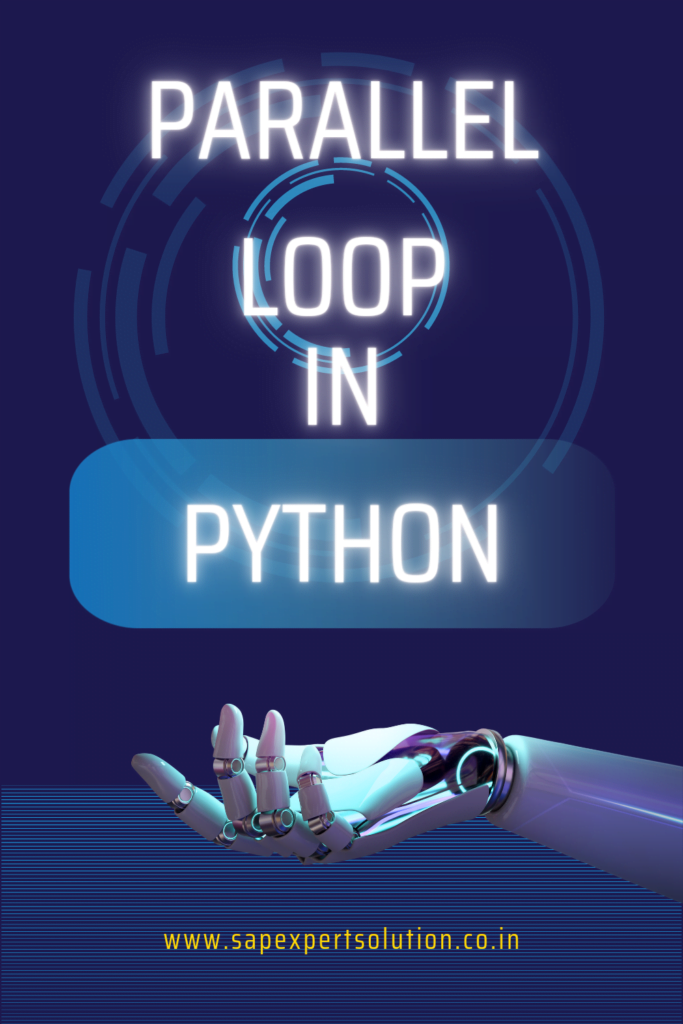
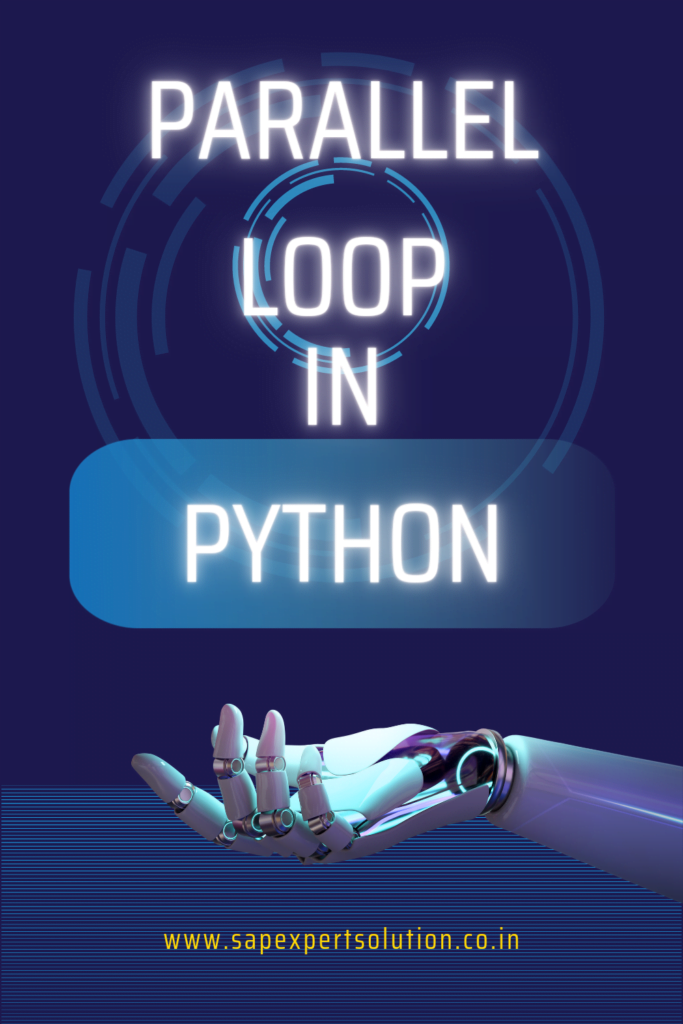
Conclusion:
In conclusion, Python arrays are a powerful tool for handling and manipulating data efficiently. With their fixed-size, homogeneous elements, and dedicated array module, they offer advantages in terms of performance and memory usage. Whether you’re working on numerical computations or memory-constrained environments, understanding and utilizing Python arrays can significantly enhance your programming capabilities.
Here are some examples :
Certainly! Here are a few examples showcasing the usage of Python arrays:
Example 1: Basic Array Creation
from array import array
# Creating an array of integers
integer_array = array('i', [1, 2, 3, 4, 5])
# Displaying the array
print("Integer Array:", integer_array)
Example 2: Array Operations
from array import array
# Creating arrays
arr1 = array('i', [1, 2, 3])
arr2 = array('i', [4, 5, 6])
# Concatenating arrays
concatenated_array = arr1 + arr2
# Displaying the concatenated array
print("Concatenated Array:", concatenated_array)
# Accessing elements
print("Element at index 2:", arr1[2])
# Slicing the array
sliced_array = arr2[1:3]
print("Sliced Array:", sliced_array)
Example 3: Numeric Computations
from array import array
# Creating numeric arrays
grades = array('f', [95.5, 89.2, 78.9, 92.3, 88.0])
# Performing computations
average_grade = sum(grades) / len(grades)
# Displaying the result
print("Average Grade:", average_grade)
Example 4: Type Safety
from array import array
# Attempting to create an array with mixed data types (will raise an error)
try:
mixed_array = array('i', [1, 2, 3.5, 4, 5])
except TypeError as e:
print(f"Error: {e}")
These examples cover basic array creation, common operations, numeric computations, and highlight the type safety aspect of Python arrays. Feel free to experiment and modify them to suit your specific use cases!
Finally, arrays in Python are an effective tool for managing and modifying data. Their memory usage and performance are enhanced by their dedicated array module and fixed-size, homogeneous elements. Whether you are working with memory-constrained environments or numerical computations, mastering Python arrays will greatly improve your programming skills.
o Examples :