Python Data types:
Python, a dynamically typed language, has several built-in data types that are important in programming. These data types allow for the storage and manipulation of various types of information. Let us look at some of the most basic data types in Python:
- Integers (
int
):
Integers represent whole numbers without any decimal point. For example:
age = 25
- Floating-Point Numbers (
float
):
Floating-point numbers represent real numbers with a decimal point or in exponential form. For example:
height = 5.9
- Strings (
str
):
Strings are sequences of characters, enclosed in single or double quotes. They are used for representing text:
name = 'Alice'
- Booleans (
bool
):
Booleans represent truth values, eitherTrue
orFalse
. They are often used in conditional statements:
is_adult = True
- Lists (
list
):
Lists are ordered, mutable sequences that can hold a variety of data types:
numbers = [1, 2, 3, 4, 5]
- Tuples (
tuple
):
Tuples are ordered, immutable sequences. Once created, their elements cannot be modified:
coordinates = (3, 5)
- Sets (
set
):
Sets are unordered collections of unique elements. They are useful for tasks like removing duplicates:
unique_numbers = {1, 2, 3, 4, 5}
- Dictionaries (
dict
):
Dictionaries are unordered collections of key-value pairs. They provide a way to store and retrieve data using unique keys:
person = {'name': 'Bob', 'age': 30, 'city': 'Paris'}
- NoneType (
None
):
TheNone
type represents the absence of a value or a null value. It is often used to signify that a variable has no assigned value.
result = None
- Complex Numbers (
complex
):
Complex numbers have a real and an imaginary part. They are expressed using thej
suffix:complex_num = 2 + 3j
Writing clear, succinct, and effective Python code requires an understanding of and effective use of these data types. By understanding when to use each type, you can make sure that your programs can accurately perform a wide range of operations on a wide range of data.
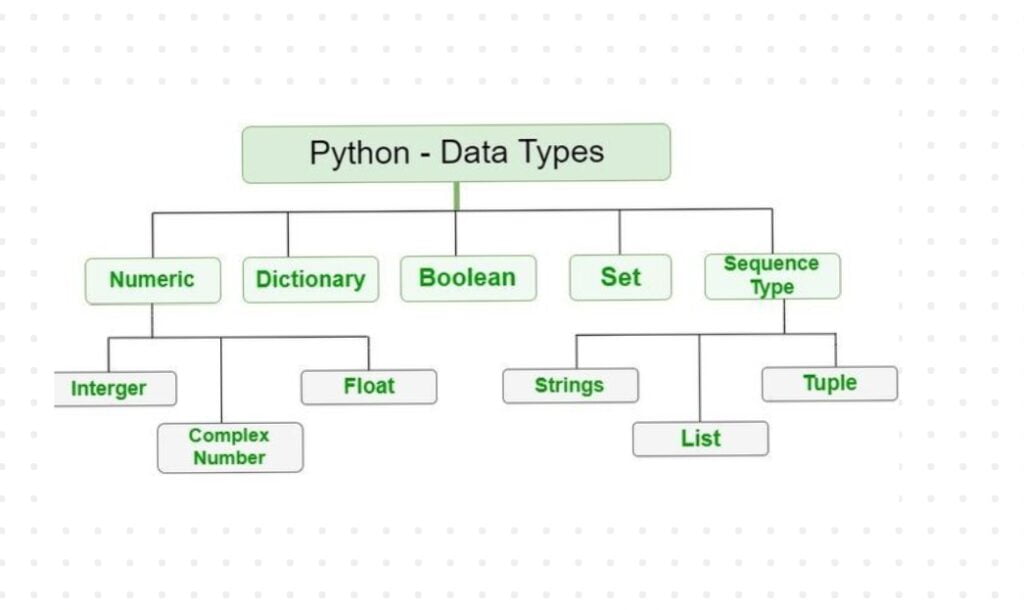
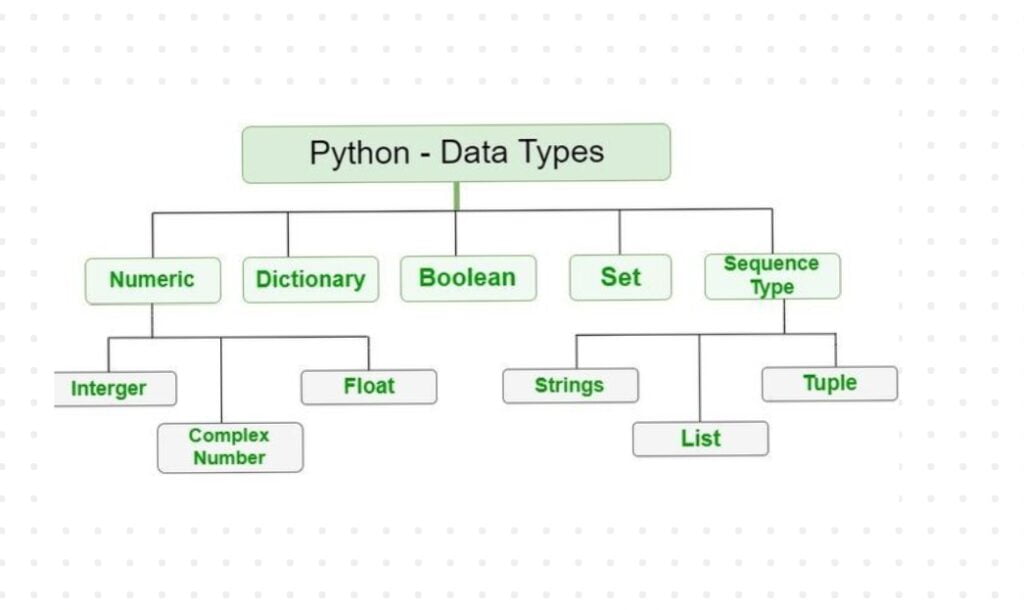
Data types in Python provide a structured way to organize and manipulate information in your programs. They offer several benefits that contribute to the efficiency, readability, and reliability of your code:
- Clarity and Readability:
Data types enhance the readability of your code by clearly specifying the kind of data a variable holds. For example, when you see a variable declared asage = 25
, it is evident thatage
is an integer. This clarity makes the code more understandable for both developers and maintainers. - Memory Efficiency:
Python’s built-in data types are optimized for memory usage. Each data type has a specific size and structure, allowing Python to allocate memory more efficiently. This optimization is particularly valuable when dealing with large datasets or memory-constrained environments. - Type Safety:
Python is a dynamically-typed language, meaning that variable types are determined at runtime. However, this dynamic typing is accompanied by a strong type system. This provides a balance between flexibility and type safety. While you can change the type of a variable, the interpreter ensures that operations are valid for the current type. - Ease of Maintenance:
Explicitly defining data types can make your code more maintainable. When working on a project or collaborating with others, having a clear understanding of the data types used helps prevent unintended errors and facilitates easier debugging and maintenance. - Built-in Operations:
Each data type comes with built-in operations and methods that simplify common tasks. For example, strings have methods for string manipulation, lists offer operations for adding or removing elements, and dictionaries provide efficient ways to access and modify key-value pairs. - Flexibility in Data Representation:
Python’s diverse set of data types allows you to represent different kinds of information in a way that makes sense for your specific use case. Whether you’re working with numerical data, text, or complex structures, Python provides the appropriate data type to handle it. - Code Optimization:
Understanding and leveraging the characteristics of specific data types can lead to optimized code. For instance, using sets for membership tests or dictionaries for efficient lookups can significantly improve the performance of your algorithms. - Compatibility and Interoperability:
Python’s built-in data types are designed to work seamlessly with various libraries and modules. This compatibility ensures that you can easily integrate third-party tools and frameworks into your projects without worrying about data type conflicts. - Error Prevention:
Using appropriate data types can help prevent common errors related to incompatible data. For example, attempting to perform arithmetic operations on strings would raise a TypeError, alerting you to a potential issue in your code.
In conclusion, data types in Python are foundational elements that contribute to the language’s versatility and ease of use. They provide a robust framework for organizing and manipulating data, making Python a powerful and expressive programming language.