The Fibonacci sequence is a fascinating mathematical concept that has captivated minds for centuries. This sequence, named after the Italian mathematician Leonardo Fibonacci, begins with 0 and 1, and each subsequent number is the sum of the two preceding ones. In this blog post, we will explore the beauty of Fibonacci sequences and various ways to implement them in Python, demonstrating the language’s elegance and versatility.
Understanding the Fibonacci Sequence: The Fibonacci sequence starts with 0 and 1, and each number after that is the sum of the two preceding numbers. It is mathematically defined by the recurrence relation (F(n) = F(n-1) + F(n-2) with seed values (F(0) = 0) and (F(1) = 1). The sequence goes as follows: 0, 1, 1, 2, 3, 5, 8, 13, 21, and so on.
Iterative Approach:
def fibonacci_iterative(n):
fib_sequence = [0, 1]
while len(fib_sequence) < n + 1:
fib_sequence.append(fib_sequence[-1] + fib_sequence[-2])
return fib_sequence[n]
The Fibonacci sequence is built iteratively by appending the sum of the last two elements at each step. While simple, it may not be the most efficient method for large values of (n) due to redundant calculations.
Recursive Approach:
def fibonacci_recursive(n):
if n <= 1:
return n
else:
return fibonacci_recursive(n - 1) + fibonacci_recursive(n - 2)
The mathematical definition of the Fibonacci sequence is directly followed by the recursive solution. However, for larger values of (n), recursion may result in redundant calculations and significant time complexity.
Memoization for Optimization:
def fibonacci_memoization(n, memo={}):
if n <= 1:
return n
elif n not in memo:
memo[n] = fibonacci_memoization(n - 1, memo) + fibonacci_memoization(n - 2, memo)
return memo[n]
Memorization is the process of storing previously computed results in order to avoid redundant calculations. A dictionary (memo) is used to store intermediate results in this implementation, significantly improving the efficiency of the recursive approach.
Dynamic Programming – Bottom-Up Approach:
def fibonacci_dynamic(n):
fib_sequence = [0, 1]
for i in range(2, n + 1):
fib_sequence.append(fib_sequence[i - 1] + fib_sequence[i - 2])
return fib_sequence[n]
Bottom-up dynamic programming builds the Fibonacci sequence iteratively from the ground up, avoiding recursion and unnecessary recalculations. For large values of (n), this method is effective and widely used.
Using Binet’s Formula for Constant Time:
import math
def fibonacci_binet(n):
sqrt_5 = math.sqrt(5)
phi = (1 + sqrt_5) / 2
psi = (1 - sqrt_5) / 2
return round((phi ** n - psi ** n) / sqrt_5)
Binet’s Formula provides a direct calculation for the (n)-th Fibonacci number in constant time. However, due to floating-point imprecision, it may produce inaccurate results for extremely large values of (n).
Visualizing Fibonacci Sequences:
import matplotlib.pyplot as plt
def plot_fibonacci_sequence(n):
fib_sequence = [0, 1]
for i in range(2, n + 1):
fib_sequence.append(fib_sequence[i - 1] + fib_sequence[i - 2])
plt.plot(range(n + 1), fib_sequence, marker='o', linestyle='-', color='b')
plt.title(f'Fibonacci Sequence up to {n}')
plt.xlabel('Index')
plt.ylabel('Fibonacci Number')
plt.grid(True)
plt.show()
Visualization is a powerful tool for understanding the progression of the Fibonacci sequence. This simple plotting function shows how each Fibonacci number evolves with increasing index.
Real-world Applications:
- Fibonacci numbers and ratios are used in financial markets to predict potential reversal or retracement levels in a price trend.
- Algorithmic Optimization: Fibonacci sequences are frequently used as test cases for algorithmic optimization. Calculating Fibonacci numbers efficiently demonstrates the effectiveness of various programming techniques.
- Pseudorandom Number Generation:
The Fibonacci sequence, when properly manipulated, can be utilized in pseudorandom number generation algorithms. - Nature and Biology:
Fibonacci sequences are found in various natural phenomena, such as the arrangement of leaves on a stem, the spirals of a pinecone, or the petals of a flower.
Conclusion:
Understanding mathematical concepts and programming paradigms is enhanced by mastering the implementation of Fibonacci sequences in Python. Whether you use iterative, recursive, or dynamic programming, each method provides information about algorithmic efficiency and optimization. As you explore the fascinating world of Fibonacci sequences, you will not only gain Python proficiency but also valuable problem-solving skills that go beyond mathematics. Accept the beauty of Fibonacci and let your Python code unravel the mysteries of this timeless sequence.
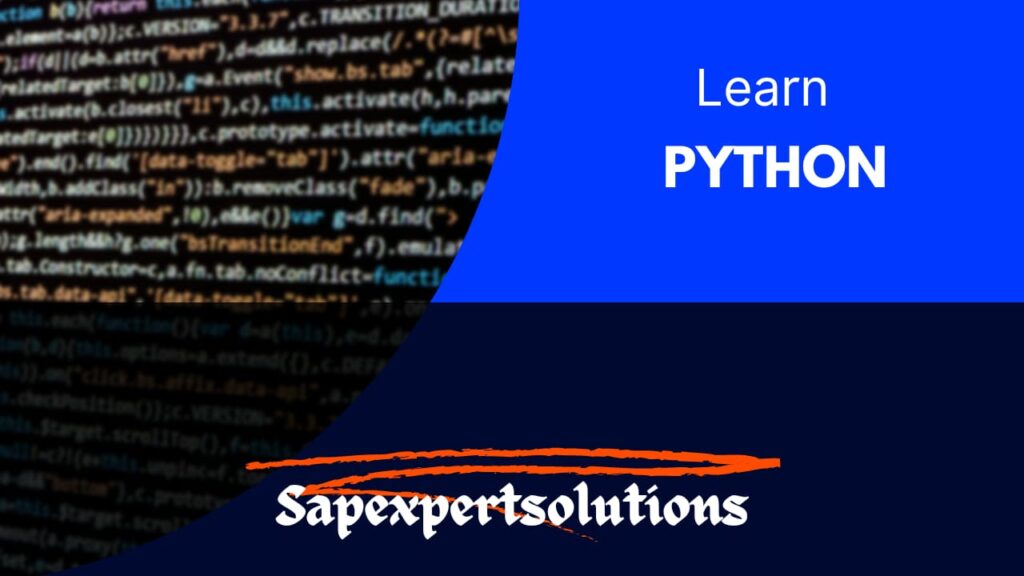
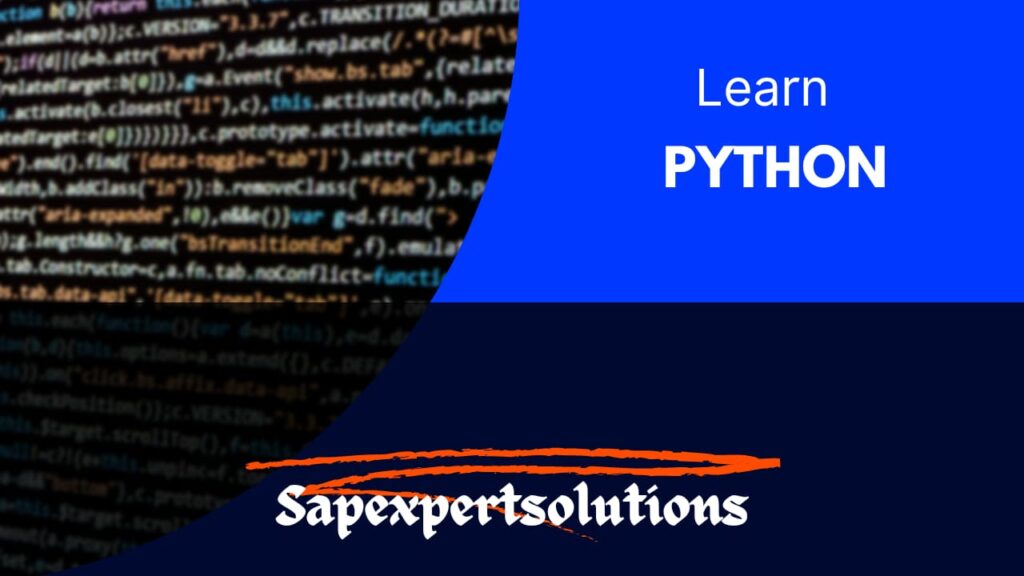
Certainly! Here are ten Python examples of Fibonacci sequence generation:
- Using a loop:
def fibonacci_loop(n):
fib_sequence = [0, 1]
while len(fib_sequence) < n:
fib_sequence.append(fib_sequence[-1] + fib_sequence[-2])
return fib_sequence[:n]
- Using recursion:
def fibonacci_recursive(n):
if n <= 1:
return n
else:
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
- Using memoization to optimize recursion:
def fibonacci_memoization(n, memo={}):
if n <= 1:
return n
elif n not in memo:
memo[n] = fibonacci_memoization(n-1, memo) + fibonacci_memoization(n-2, memo)
return memo[n]
- Using generators:
def fibonacci_generator(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
- Using list comprehension:
def fibonacci_list_comprehension(n):
fib_sequence = [0, 1] + [fib_sequence[i-1] + fib_sequence[i-2] for i in range(2, n)]
return fib_sequence[:n]
- Using reduce function:
from functools import reduce
def fibonacci_reduce(n):
fib_sequence = reduce(lambda x, _: x + [x[-2] + x[-1]], range(n-1), [0, 1])
return fib_sequence[:n]
- Using matrix exponentiation:
import numpy as np
def fibonacci_matrix_exponentiation(n):
matrix = np.array([[1, 1], [1, 0]])
result = np.linalg.matrix_power(matrix, n - 1)
return result[0, 0]
- Using a closed-form formula:
import math
def fibonacci_closed_form(n):
sqrt_5 = math.sqrt(5)
phi = (1 + sqrt_5) / 2
return round((phi ** n) / sqrt_5)
- Using itertools accumulate:
from itertools import accumulate, repeat
def fibonacci_accumulate(n):
fib_sequence = list(accumulate(repeat(1), lambda x, _: x + [x[-2] + x[-1]], initial=[0, 1]))
return fib_sequence[:n]
- Using Binet’s Formula:
import math
def fibonacci_binet(n):
sqrt_5 = math.sqrt(5)
phi = (1 + sqrt_5) / 2
psi = (1 - sqrt_5) / 2
return round((phi**n - psi**n) / sqrt_5)
Choose the method that best meets your needs or experiment with different approaches!